CI/CD Tools for Cloud Applications on Kubernetes
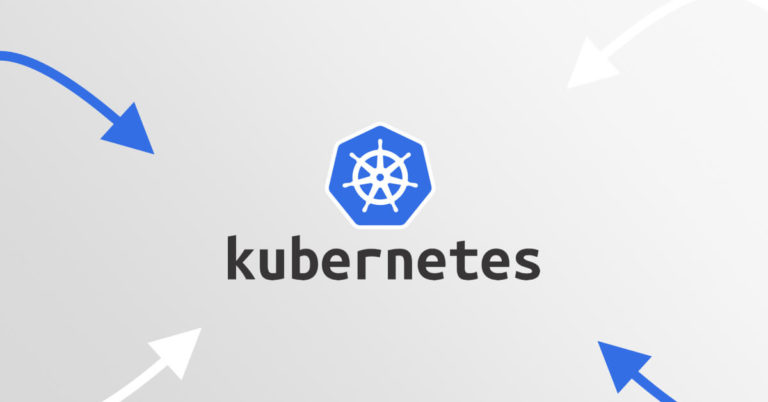
Kubernetes is the de facto industry standard for container management and orchestration. Not surprisingly, it has also become common to use Kubernetes in tandem with compatible Continuous Integration (CI) and Continuous Deployment (CD) tools.More on the subject:
As a container orchestrator, Kubernetes expects deployments to spin up software throughout a cluster. Those deployments are created using files or command lines that can be generated manually or using properly configured CI/CD software.
CI/CD for Kubernetes
Continuous Integration dictates that every code change must be tested in a way that makes it possible to detect bugs before the software is deployed to production or staging. With Continuous Deployment, every successful code change is ready to be deployed. Developers are so confident about their tests that code changes can be deployed to production automatically.
Even if tests fail to detect a bug and the code goes to production with it, there are ways to avoid a serious issue. First, proper monitoring and logging tools can identify bugs. Second, a streamlined deployment process can roll out the new version of the code, slowing changing older instances for new ones.
Kubernetes makes continuous deployment much more manageable by automating the deployment process. Since Kubernetes only runs container images, the software and its dependencies are already deployed into the container image, without the need to install dependencies first. Moreover, since Kubernetes manages how each machine in the cluster allocates its resources, the best place to put the new version of the software is decided automatically. Finally, rolling updates are natural and native on Kubernetes.
On Kubernetes, a deployment is a unit that describes how a Pod will be deployed. A pod can contain one or more containers that must be described with the same document. The containers inside a Pod share storage and network, behaving much like a Docker Compose file.
apiVersion: apps/v1 kind: Deployment metadata: name: logz-tutorial spec: replicas: 3 template: metadata: labels: app: logz-tutorial spec: containers: - name: logz-tutorial image: logz-tutorial:1 imagePullPolicy: Always ports: - containerPort: 80 livenessProbe: httpGet: path: /health port: 8443 scheme: HTTPS initialDelaySeconds: 30 timeoutSeconds: 3 periodSeconds: 10 successThreshold: 1 failureThreshold: 3
In the example above, an image called logz-tutorial, version 1 with three replicas is being deployed. If the logz-tutorial upgrades to version 2, the file above needs to be changed or a command line must be issued. Kubernetes will take care of the entire deployment process using a rolling update technique. First, it will deploy a new pod with the new version and remove the one with the older version, continuing until all the pods deployed match the specified version. A human or computer operator can stop the rolling process if an issue is detected.
Although Kubernetes, like many other container orchestrators, simplifies the deployment process, there are still some files and command lines that need to be learned. However, even they can be automated, using the right tools. These tools fall into three different categories:
- CI Tools—These are tools that run continuous integration tasks. It is possible to deploy them on Kubernetes using custom tasks or plugins, though the process is not as smooth as it is for a CD Tool.
- CD Tools—CD tools are specialized to deploy artifacts into environments. Generally, they are triggered by a CI tool after validation.
- Package Managers—Just as APT is a package tool for Debian-based distributions and Docker is a package tool for containers, there are some tools that create packages for Kubernetes. The user just needs to download the package and deploy it on the cluster, with minor intervention.
CI Tools
Jenkins
Jenkins is a self-contained, open-source automation server that achieves continuous integration and build automation. Jenkins is built into Java and provides many plugins to support building, testing, deploying, and automating a project. It can be installed through a native system, Docker, or it can run in a Java environment. Jenkins can also be extended by extra plug-ins such as the Kubernetes plugin, which allows easy deployment using pipelines.
Travis CI
Travis CI is only free to open-source, Github hosted projects, but it is quite popular because it makes running new pipelines effortless. While Jenkins is a self-hosted service, Travis is a CI-as-a-service, which means it is hosted by and runs in a third party server.
Currently, there are two ways to deploy Travis CI on Kubernetes. One requires creating a new pipeline job and deploying the service using the Kubectl command line. The other involves using a terraform recipe. Neither of these methods is refined at the moment.
GitLab Community Edition
GitLab is a full-featured software development platform that includes GitLab CI/CD to leverage the capacity to build, test, and deploy without the requirement to integrate with external tools. Developers can use one single application in all stages of the work cycle of the same product (product, development, QA, security, and operations).
GitLab also has integration with Kubernetes. With this integration, you can monitor the cluster and control the deployment of every artifact. A feature called Auto DevOps can create an entire CI/CD pipeline based on your technology stack. In the end, GitLab will deploy the application automatically on your Kubernetes Cluster.
Gitlab has a free open-source version that supports Kubernetes integration. Paid versions increase this support to more advanced enterprise-oriented features.
CD Tools
Spinnaker
Spinnaker is an open-source continuous deployment tool initially developed by Netflix. It supports Kubernetes with an official provider which integrates with Spinnaker. Once configured, Spinnaker reads the entire cluster and shows all the applications that are already available. With Spinnaker, it is possible to create deployment pipelines that can be triggered by a CI tool or a new Docker image. Once Kubernetes is configured, deploying a new service in the cluster is straightforward.
Weave Cloud
While Spinnaker is a generic CD platform with Kubernetes as one of its options, Weave Cloud is more Kubernetes-centric. It provides continuous delivery and allows monitoring and performance diagnostics, but it is not free.
Logz.io Apollo
Logz.io Apollo takes a different approach towards CD with Kubernetes. It is designed to simplify deployment and integration with other tools, such as CI tools. To implement it, all you need to do is notify Apollo that the artifact is ready to be deployed. Moreover, Apollo has monitoring and debugging features and a straightforward installation process that applies updates on its own.
Apollo can manage more than one cluster at a time and has fine-grained user permissions. This means that each user deploys only what they need where they need it. For example, QA teams may only have access to the staging environment, while DevOps teams must have broader permissions.
Package Managers
Helm
Not all software can fit into a single container. Many applications rely on a different set of services. Even a single application may need more than a single container (the backend of a database, for example), while a complex application needs dozens of services integrated with cloud resources like load balancers or shared storages.
Helm is a package manager capable of deploying as many services and cloud resources as an application needs. Helm also has a curated list of high-quality packages (called “charts” in the Helm universe) that can be deployed on Kubernetes. Many of the tools described below have a chart on this list.
Below is an example of how easy it is to deploy a new application on Kubernetes using Helm:
This command line
> helm install stable/redis-ha
installs Redis Cache in High Availability mode. This means three pods are going to be installed: one containing Redis master and a sentinel container, and the other two pods containing slave units of Redis. More information about this chart can be seen here.
Draft
Draft is not an alternative to Helm; it is a complementary tool. It uses Helm internally to facilitate container development. With Draft, a user can develop an application without any container or Kubernetes awareness, run simple commands, and create packages ready to be deployed into the orchestrator.
Once configured, the developer needs to issue the command below on the application directory to create an artifact:
> draft create
Once the package is created, another command will containerize and deploy on Kubernetes:
> draft up
Draft auto-detects the technology stack and applies the correct procedures for deployment. A list of officially available stacks can be found here.
Draft works smoothly with well-defined projects, but it does not operate as well within legacy systems or unusual technology stacks. Draft is currently in a preview state, so additional developments may fix these problems.
Conclusion
In recent years, Continuous Integration and Continuous Delivery have become familiar to every software engineer and developer. These days, it’s almost impossible to create new software without a proper CI/CD pipeline.
With the popularity of Kubernetes, even mature tools are developing new features to integrate with Kubernetes. Because Kubernetes is the dominant solution for container orchestration, developers have started trying to improve and smooth the deployment process on every CI/CD tool available.
This article explored some popular tools for deploying new artifacts into Kubernetes. With the wide array of options available, you should be able to fit Kubernetes deployment right on your stack.
Get started for free
Completely free for 14 days, no strings attached.